<값1>이 Map, List, 배열일 경우 1. <값1>이 Map일 경우 1. <값1>.containKey(<값2>)이 false이면 null 리턴 2. 아니면 <값1>.get(<값2>) 리턴 2. <값1>이 List, 배열일 경우 1. <값2>가 정수값인지 여부를 겁사(정수가 아니면 에러 발생) 2. <값1>.get(<값2>) or Arrays.get(<값1>, <값2>) 결과를 리턴 3. 익셉션을 발생하면 에러를 발생 값2>값1>값2>값1>값2>값1>값2>값1>값2>값1>값1>값1>
<값1>이 다른 객체일 경우 1. <값2>를 문자열로 변환 2. <값1>객체가 <값2>를 이름으로 갖는 읽기 가능한 프로퍼티를 포함하고 있다면 프로퍼티 값을 리턴 3. 그렇지 않을 경우 에러발생 값2>값1>값2>값1>
### EL Object Navigation page, request, session, application 영역에 저장된 속성에 접근할 때에는 pageScope, requestScope, sessionScope, applicationScope 기본객체를 사용 page영역에 저장되어 있는 NAME 속성 참고하기 {: .label .label-purple .mt-2} ```jsp ${pageScope.NAME} ``` ### Operator of EL 1. 수치 연산자 : +, -, *, /, % ```jsp ${"10" + 1} 결과는 11 (자바는 101) ${"일" + 1} 결과는 에러발생 (자바는 일1) ${null + 1} 결과는 1 // null이면 0을 리턴함 ``` 2. 비교 연산자 : ==, !=, <, <=, >, >= 3. 논리 연산자 : &&, ||, ! 4. empty연산자 : empty<값>의 형태로 사용 null, "", 길이 0인 배열, 빈 Map, 빈 Collection = true 5. 삼항연산자 : 수식 ? 값1 : 값2 6. 문자열 연결 : += ```jsp ${"문자" += "열" += "연결"} //"문자열연결" <% request.setAttribute("title","JSP 프로그래밍"); %> ${ "제목: " += title} //제목: JSP 프로그래밍 ``` 7. 세미콜론(;) 연산자 ${1+1; 10+10}의 결과는 20만 출력 즉 ${A;B}의 EL결과는 B값만 출력 8. 할당연산자 할당 연산자 자체도 출력 결과를 생성하기 때문에 세미콜론 연산자와 같이 활용해서 사용함 ```jsp ${var = 10} //10으로 출력 ${hangul = ['가','나','다']; "} //응답결과에 빈 문자열 출력 ${hangul[0]} //['가','나','다'] 사용가능 ``` 9. 특수문자 처리하기 역슬래쉬 뒤에 문자를 위치시키면 됨 --- ## EL's Method Call ### Thermometer.java 섭씨를 화씨로변경하는 온도계 객체 생성 {: .label .label-purple .mt-2} ```java package com.lec.el; import java.util.HashMap; import java.util.Map; public class 온도계 { private Map<String, Double> map = new HashMap<>(); public String getInfo() { return "섭씨/화씨온도 변환기"; } public void setCelsius(String city, Double val) { map.put(city, val); } public Double getCelsius(String city) { return map.get(city); } public Double getFahrenheit(String city) { Double celsius = getCelsius(city); if(celsius==null) return null; return celsius.doubleValue() * 1.8 + 32.0; } } ``` 생성한 온도계 자바클래스 사용하기 {: .label .label-purple .mt-2} ```jsp <%@page import="com.lec.el.온도계"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <% 온도계 thermometer = new 온도계(); request.setAttribute("t", thermometer); %> <!DOCTYPE html>
온도변환기(v1.0) ``` 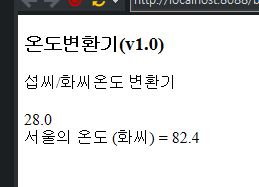 ### Call Static Method 1 정적 메서드를 호출하는 방법 1 : EL의 함수로 지정하는 방법 TLD 파일작성, web.xml파일설정, taglib 디렉티브도 설정, 호출 TLD파일에 설정한 이름을 사용 등... ### Call Static Method 2 정적 메서드를 호출하는 방법 2 : EL 3.0이후 추가됨 static 메서드 생성하기 {: .label .label-purple .mt-2} ```java package com.lec.el; import java.text.DecimalFormat; public class FormatUtil { public static String number(long number, String pattern) { DecimalFormat ptrn = new DecimalFormat(pattern); return ptrn.format(number); } } ``` 메서드를 호출하기 위한 JSP 코드 작성 {: .label .label-purple .mt-2} ```jsp <%@page import="com.lec.el.FormatUtil"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <% request.setAttribute("price", 1230l); %> <!DOCTYPE html>
정적메서드 호출하기 ```  --- ## Disable EL EL을 비활성화 시키는 두가지 방법 ### web.xml web.xml파일에서 EL을 비활성화 시켜주는 옵션을 추가하기 web.xml파일: EL을 비활성화 {: .label .label-purple .mt-2} ```xml /oldversion/*true ``` web.xml파일: #{expr}만 비활성화 {: .label .label-purple .mt-2} ```xml /oldversion/*true/oldversion2_4/* true ``` ### Specify deactivation options in JSP Page 1. isELIgnored : ture일 경우 EL을 일반 문자열로 처리 2. deferredSyntaxAllowedAsLiteral : 이 값이 treu일 경우 #{expr} 형식의 EL을 문자열로 처리 ```jsp <%-- EL을 비활성하 시키는 경우 --%> <%@ page isELIgnored="true" %> <%-- #{expr} 형식의 EL을 비활성화 시키는 경우 --%> <%@ page deferredSyntaxAllowedAsLiteral="true" %> ``` 값>표현>표현>표현>